Node.js
Node.js is a runtime environment that allows you to run JavaScript on the server-side. It's lightweight, scalable, and perfect for building fast, data-intensive applications.
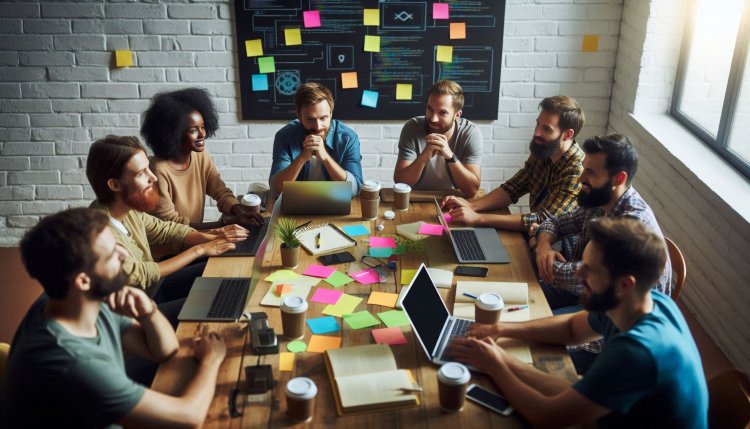
Node.js
Node.js is an open-source, cross-platform JavaScript runtime environment that allows developers to run JavaScript code outside of a web browser. It is built on Chrome's V8 JavaScript engine and uses an event-driven, non-blocking I/O model that makes it lightweight and efficient for building scalable network applications.
Features of Node.js
- Asynchronous and Event-Driven: Node.js uses asynchronous programming, allowing multiple operations to be performed concurrently without blocking the execution of the program. It also follows an event-driven architecture, where certain actions trigger events that can be handled by event listeners.
- Single-Threaded, Non-Blocking: Node.js operates on a single thread using non-blocking I/O calls, which means that it can handle multiple connections simultaneously without creating a new thread for each request. This makes Node.js highly efficient and suitable for real-time applications.
- Extensive Package Ecosystem: Node.js has a rich ecosystem of libraries and modules available through npm (Node Package Manager), which allows developers to easily extend the functionality of their applications by integrating third-party packages.
- Fast Execution: Node.js is known for its high performance and fast execution speed, thanks to the V8 engine. This makes it ideal for building server-side applications that require quick response times.
- Scalability: Node.js is designed to be scalable, allowing developers to handle large numbers of concurrent connections with ease. It can be used to build scalable network applications that can handle heavy loads without compromising performance.
Common Use Cases
Node.js is widely used for various types of applications, including:
- Web Applications: Node.js is commonly used to build web applications, including APIs, server-side web applications, and real-time applications like chat applications and online gaming platforms.
- Microservices: Node.js is well-suited for building microservices architecture, where applications are broken down into smaller, independent services that communicate with each other through APIs.
- IoT (Internet of Things) Applications: Node.js is often used in IoT applications due to its lightweight nature and ability to handle real-time data processing.
- Data Streaming Applications: Node.js is ideal for building applications that require data streaming, such as video and audio streaming services.
Getting Started with Node.js
To get started with Node.js, you will need to install Node.js on your machine. You can download the installer from the official Node.js website (https://nodejs.org/) and follow the installation instructions for your operating system.
Creating a Simple Node.js Application
Once you have Node.js installed, you can create a simple Node.js application by following these steps:
- Create a new directory for your project and navigate into it.
- Run
npm init
to initialize a new Node.js project. This will create apackage.json
file that contains information about your project. - Create a new JavaScript file (e.g.,
app.js
) and write your Node.js code in it. For example:
```javascript const http = require('http'); const server = http.createServer((req, res) => { res.writeHead(200, { 'Content-Type': 'text/plain' }); res.end('Hello, World!'); }); server.listen(3000, 'localhost', () => { console.log('Server running at http://localhost:3000/'); }); ```
Save the file and run it using Node.js by executing node app.js
in the terminal. This will start a simple HTTP server that listens on port 3000 and responds with "Hello, World!" to incoming requests.
Conclusion
Node.js is a powerful and versatile runtime environment that allows developers to build fast, scalable, and efficient server-side applications using JavaScript. Its asynchronous and event-driven architecture, along with its extensive package ecosystem, make it a popular choice for a wide range of applications.
What's Your Reaction?
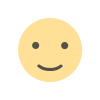
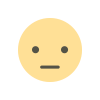
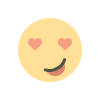
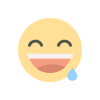
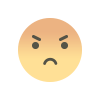
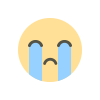
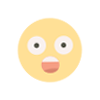