Spring Framework
The Spring Framework is a powerful and comprehensive tool for building robust Java applications. Learn more about its features and benefits.
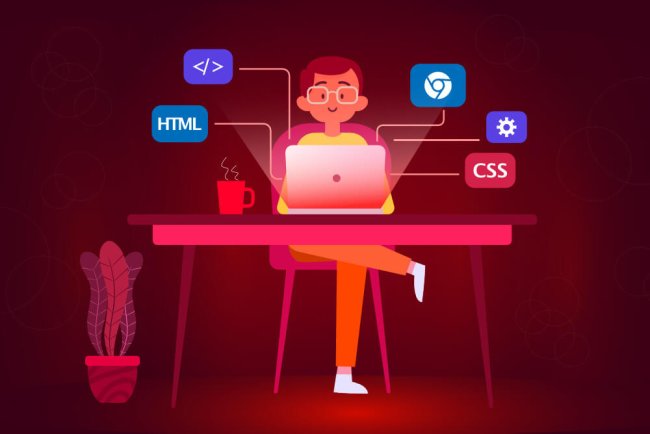
Spring Framework
The Spring Framework is a powerful and comprehensive framework for building modern Java applications. It provides a wide range of features and capabilities that help developers create robust, scalable, and maintainable applications.
Core Features
- Inversion of Control (IoC): One of the key features of the Spring Framework is its support for Inversion of Control. IoC allows developers to make their code more modular and decoupled by removing the responsibility of object creation and lifecycle management from the application code.
- Dependency Injection (DI): Spring uses Dependency Injection to inject the required dependencies into a class rather than having the class create the dependencies itself. This promotes loose coupling between classes and makes it easier to test and maintain the code.
- Aspect-Oriented Programming (AOP): AOP is a programming paradigm that allows developers to separate cross-cutting concerns, such as logging, security, and transaction management, from the core business logic of an application. Spring provides powerful support for AOP through its AspectJ integration.
- Transaction Management: Spring offers comprehensive support for managing transactions in Java applications. It provides declarative transaction management through annotations or XML configuration, as well as programmatic transaction support.
Modules
The Spring Framework is organized into several modules, each providing specific functionality to address different aspects of application development. Some of the core modules include:
- Spring Core Container: This module provides the foundational building blocks of the Spring Framework, including IoC and DI features.
- Spring AOP: This module offers support for Aspect-Oriented Programming, allowing developers to separate concerns such as logging and security from the core application logic.
- Spring JDBC: This module simplifies database access in Java applications by providing a higher-level abstraction over JDBC API.
- Spring ORM: This module provides integration with popular Object-Relational Mapping (ORM) frameworks such as Hibernate and JPA, simplifying database operations with object-oriented models.
- Spring Web: This module offers features for building web applications using Spring, including support for MVC architecture, RESTful services, and integration with popular web frameworks like Spring Boot.
Spring Boot
Spring Boot is an extension of the Spring Framework that simplifies the process of building production-ready applications. It provides a set of conventions and auto-configuration features that reduce the amount of boilerplate code required in a Spring application.
- Auto-Configuration: Spring Boot automatically configures the application based on the dependencies present in the classpath. This eliminates the need for manual configuration, making it easier to get started with Spring applications.
- Embedded Servers: Spring Boot comes with embedded servers such as Tomcat, Jetty, and Undertow, allowing developers to package their applications as standalone executable JAR files.
- Actuator: Spring Boot Actuator provides production-ready features for monitoring and managing Spring applications, including health checks, metrics, and environment information.
Spring Security
Spring Security is a powerful authentication and authorization framework for securing Java applications. It provides a wide range of features for implementing security requirements, such as user authentication, access control, and protection against common security vulnerabilities.
- Authentication: Spring Security offers support for various authentication mechanisms, including form-based authentication, HTTP Basic authentication, and OAuth.
- Authorization: Developers can define access control rules using annotations or configuration to restrict access to specific resources based on user roles or permissions.
- CSRF Protection: Spring Security provides built-in protection against Cross-Site Request Forgery (CSRF) attacks by generating and validating CSRF tokens in web applications.
What's Your Reaction?
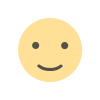
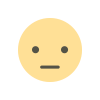
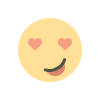
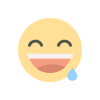
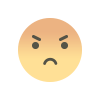
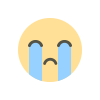
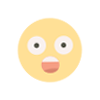