Softmax Function
Discover the mathematical formula behind the Softmax function, a popular choice for classification problems in machine learning.
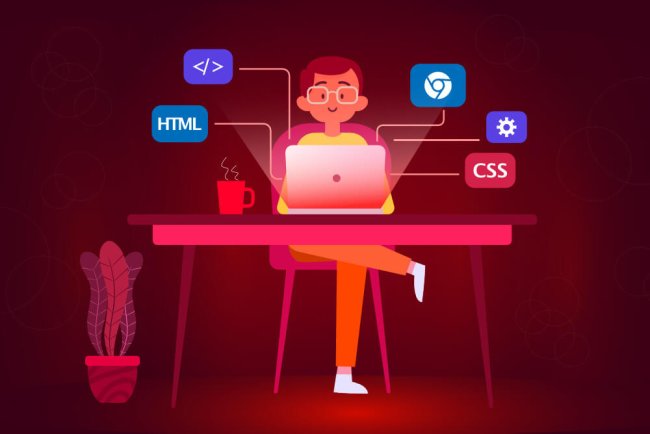
Softmax Function
The softmax function is a commonly used activation function in neural networks, especially in the output layer of a classification model. It converts raw scores or logits into probabilities that sum up to 1. This allows the model to output a probability distribution over multiple classes, making it suitable for multi-class classification tasks.
Mathematical Representation
The softmax function takes a vector of K real numbers as input and outputs a vector of the same size, where each element is in the range (0, 1) and the sum of all elements is 1. The softmax function is defined as:
$$softmax(x)_i = \frac{e^{x_i}}{\sum_{j=1}^{K} e^{x_j}}$$
where \(x\) is the input vector of logits or raw scores, \(i\) is the index of the element in the output vector, and \(K\) is the number of classes. The softmax function exponentiates each element of the input vector and then normalizes the values to obtain a probability distribution.
Properties of Softmax Function
- Normalization: The softmax function ensures that the output probabilities sum up to 1, making it suitable for classification tasks.
- Monotonicity: As the input scores increase, the corresponding probabilities also increase, but in a normalized manner.
- Sensitivity: The softmax function amplifies the differences between the input scores, making it easier to distinguish between classes.
- Non-linearity: The softmax function introduces non-linearity, allowing neural networks to learn complex patterns and relationships.
Implementation in Python
Here is a simple implementation of the softmax function in Python:
import numpy as np
def softmax(x):
exp_scores = np.exp(x)
probs = exp_scores / np.sum(exp_scores, axis=1, keepdims=True)
return probs
# Test the softmax function
x = np.array([[1.0, 2.0, 3.0],
[0.5, 1.0, 2.0]])
print(softmax(x))
In this implementation, we first exponentiate the input scores using NumPy's exp
function. Then, we compute the softmax probabilities by dividing the exponentiated scores by the sum of all exponentiated scores along the appropriate axis (usually axis=1 for row-wise normalization).
Applications of Softmax Function
The softmax function is widely used in various machine learning tasks, including:
- Image Classification: Softmax is commonly used in neural networks for classifying images into multiple categories.
- Natural Language Processing: Softmax is used in language models for predicting the next word in a sequence.
- Reinforcement Learning: Softmax is used in policy gradient methods for selecting actions based on probabilities.
Limitations of Softmax Function
While the softmax function is effective for multi-class classification, it has some limitations:
- Vanishing Gradient: The gradients of the softmax function can vanish when the input scores are very large or very small, leading to slow convergence during training.
- Label Smoothing: Softmax tends to produce confident predictions, which may not reflect the uncertainty in the model. Label smoothing techniques are often used to address this issue.
- Class Imbalance: Softmax assumes that the classes are mutually exclusive, which may not hold in some real-world scenarios with imbalanced data.
What's Your Reaction?
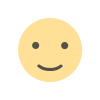
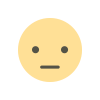
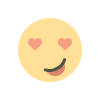
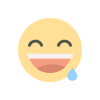
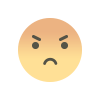
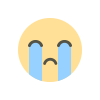
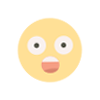