React.js
Learn React.js, a popular JavaScript library for building user interfaces. Discover its component-based architecture and efficient virtual DOM rendering.
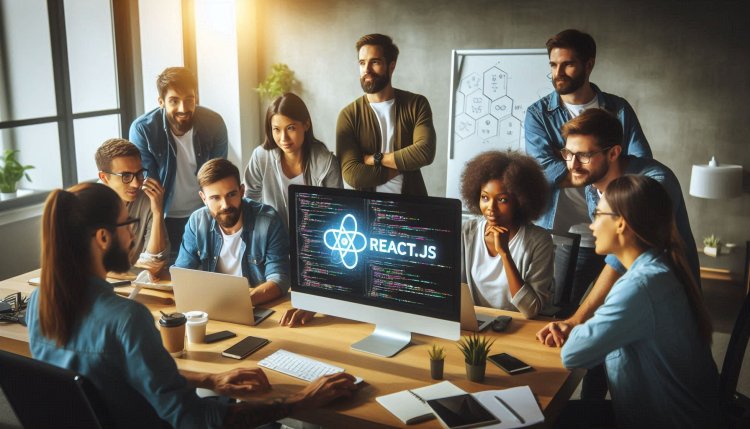
React.js: A Comprehensive Guide
React.js is a popular JavaScript library for building user interfaces. It was developed by Facebook and released in 2013. React.js is known for its declarative and component-based approach to building UIs, making it easier to create interactive and dynamic web applications.
Key Concepts
Components
In React, everything is a component. A component is a reusable piece of code that represents a part of the user interface. Components can be simple, like a button, or complex, like an entire form. Components can also be composed of other components, allowing for a modular and scalable approach to building UIs.
Virtual DOM
React uses a Virtual DOM to efficiently update the user interface. When the state of a component changes, React updates the Virtual DOM with the new state. React then compares the Virtual DOM with the actual DOM and only applies the necessary changes, resulting in faster and more efficient updates.
JSX
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript files. JSX makes it easier to define the structure of your components and is compiled to standard JavaScript by tools like Babel. JSX is not required to use React, but it is commonly used in React applications for its readability and convenience.
How React Works
When you build a React application, you define components that represent different parts of the UI. Each component manages its own state and handles user interactions. When the state of a component changes, React re-renders the component and its children, updating the UI to reflect the new state.
React uses a unidirectional data flow, where data flows from parent components to child components through props. This makes it easier to manage the state of your application and ensures that changes in one part of the UI do not affect other parts unintentionally.
Getting Started with React
To start building with React, you can use tools like Create React App, which sets up a new React project with all the necessary configurations and dependencies. Once you have set up your project, you can start creating components, defining their structure and behavior, and composing them to build your application.
Example
Here is a simple example of a React component that displays a greeting message:
```jsx import React from 'react'; function Greeting({ name }) { return
Hello, {name}!
; } export default Greeting; ```
In this example, we have defined a functional component called `Greeting` that takes a `name` prop and displays a greeting message. We can use this component in other parts of our application by importing and rendering it.
Benefits of React
Declarative
React allows you to describe how your UI should look at any given time, rather than imperatively manipulating the DOM. This makes it easier to reason about your code and maintain consistency in your application's state.
Component-Based
React's component-based architecture promotes reusability and modularity. You can create components that encapsulate specific functionality and reuse them throughout your application. This makes it easier to maintain and scale your codebase as your application grows.
Efficient Updates
React's Virtual DOM and diffing algorithm make updates to the UI more efficient. React only updates the parts of the UI that have changed, resulting in faster rendering and better performance, especially in complex applications with many components.
Common Patterns in React
State Management
React components can have local state that is managed internally by the component itself. You can update the state of a component using the `useState` hook or by extending the `Component` class in class components. State management is essential for building interactive and dynamic user interfaces.
Props
Props are used to pass data from parent components to child components in React. Props are immutable and are used to configure the behavior and appearance of a component. By passing props to components, you can create reusable and composable UI elements.
Handling Events
In React, you can handle user interactions by attaching event handlers to DOM elements. Event handlers are functions that are called when a specific event occurs, such as a button click or form submission.
What's Your Reaction?
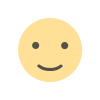
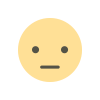
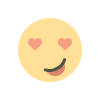
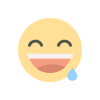
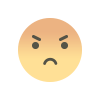
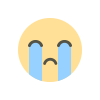
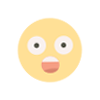