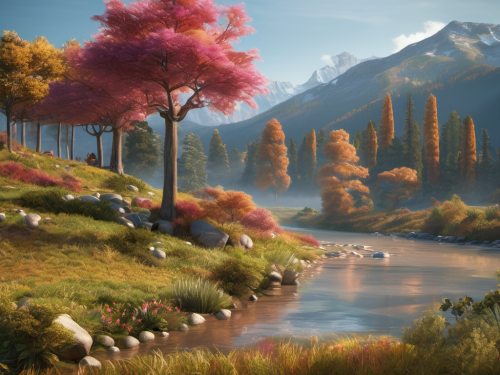
K-Nearest Neighbors (KNN) is a simple and effective supervised machine learning algorithm used for classification and regression tasks. It is a non-parametric and lazy learning algorithm, meaning it does not make assumptions about the underlying data distribution and does not learn a specific model during training. Instead, KNN stores all available data points and classifies new data points based on the majority class of their k nearest neighbors in the feature space.
The key idea behind the KNN algorithm is to predict the class of a new data point by looking at the majority class of its k nearest neighbors. The distance metric used to measure the similarity between data points is typically Euclidean distance, but other distance metrics like Manhattan distance or cosine similarity can also be used based on the nature of the data.
When a new data point is to be classified, KNN calculates the distances to all other data points in the training set and selects the k nearest neighbors based on the distance metric. The class label of the new data point is then determined by a majority vote among its k nearest neighbors. In the case of regression tasks, the output is the average of the k nearest neighbors' target values.
One of the critical hyperparameters in the KNN algorithm is the value of k, which determines the number of nearest neighbors considered for classification. The choice of k can significantly impact the algorithm's performance, as a small k value can lead to overfitting and a large k value can lead to underfitting.
Choosing an optimal value of k often involves using techniques like cross-validation to evaluate the model's performance for different values of k and selecting the value that provides the best balance between bias and variance. It is essential to experiment with different values of k to find the most suitable one for the dataset at hand.
K-Nearest Neighbors has been successfully applied in various fields, including:
Here is an example of implementing K-Nearest Neighbors algorithm in Python using the scikit-learn library:
```python from sklearn.neighbors import KNeighborsClassifier from sklearn.model_selection import train_test_split from sklearn.datasets import load_iris # Load the Iris dataset iris = load_iris() X = iris.data y = iris.target # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create a KNN classifier knn = KNeighborsClassifier(n_neighbors=3) # Fit the model on the training data knn.fit(X_train, y_train) # Make predictions on the test data predictions = knn.predict(X_test) # Evaluate the model accuracy = knn.score(X_test, y_test) print("Accuracy: {:.2f}".format(accuracy)) ```