Exponential Linear Unit (ELU)
Learn about Exponential Linear Unit (ELU) activation function in neural networks. Understand its benefits and how it can improve model performance.
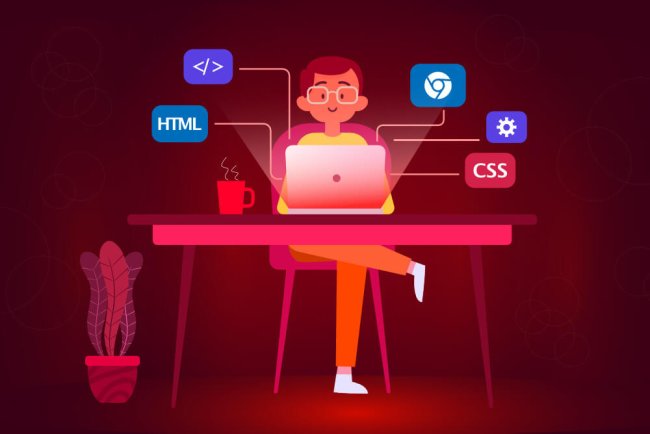
Exponential Linear Unit (ELU)
The Exponential Linear Unit (ELU) is an activation function commonly used in artificial neural networks. It is a variant of the Rectified Linear Unit (ReLU) function and was introduced to address some of the limitations of ReLU. ELU has gained popularity in deep learning due to its ability to handle the vanishing gradient problem and its improved performance in certain cases.
Mathematical Formulation
The ELU function is defined as:
ELU(x) = x if x >= 0
ELU(x) = α * (exp(x) - 1) if x < 0
Where α is a hyperparameter that controls the output of the function for negative values of x. Typically, α is set to a small positive value such as 1.0.
Properties of ELU
- Range: ELU produces output values in the range (-∞, ∞).
- Smoothness: ELU is smooth and continuously differentiable, which can help in gradient-based optimization.
- Zero-centered: ELU is zero-centered, which can help in optimizing the weights of neural networks.
- Handle vanishing gradient problem: ELU helps in mitigating the vanishing gradient problem by allowing negative values, unlike ReLU which sets negative values to zero.
Comparison with ReLU
While ReLU is a popular activation function in deep learning due to its simplicity and computational efficiency, it suffers from the problem of "dying ReLU neurons" where neurons can become inactive and stop learning during training. ELU addresses this issue by allowing negative values, which helps neurons to adapt and continue learning even for negative inputs.
Another advantage of ELU over ReLU is that it is zero-centered, which can help in optimizing the weights of the neural network. Zero-centered activations tend to have a more symmetric effect on the weights, making it easier for the optimizer to update them during training.
Implementation in Python
Here is a simple implementation of the ELU activation function in Python:
import numpy as np
def elu(x, alpha=1.0):
return np.where(x >= 0, x, alpha * (np.exp(x) - 1))
# Example usage
x = np.array([-2, -1, 0, 1, 2])
print(elu(x))
In this implementation, the function takes an input array x and an optional parameter alpha (default value is 1.0) and applies the ELU function element-wise to the input array.
Applications of ELU
ELU can be used in various deep learning architectures, including convolutional neural networks (CNNs), recurrent neural networks (RNNs), and feedforward neural networks. It is particularly useful in scenarios where the vanishing gradient problem is a concern, as ELU's ability to handle negative values can help in improving the learning process.
ELU has been shown to perform well in tasks such as image recognition, natural language processing, and speech recognition. Its smoothness and zero-centered nature make it a suitable choice for optimizing neural networks and achieving better convergence during training.
What's Your Reaction?
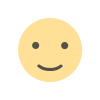
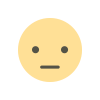
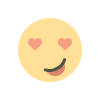
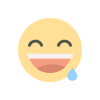
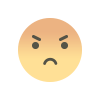
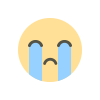
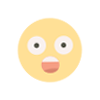