Cross-Validation
Cross-validation is a statistical method used to evaluate the performance and generalizability of machine learning models. Learn its importance and how to implement it.
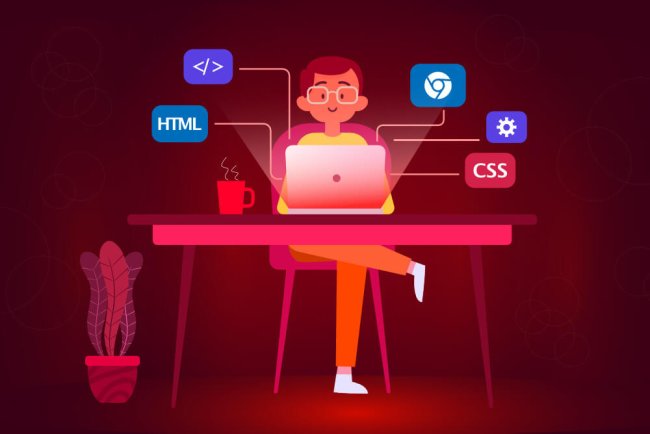
Cross-Validation
Cross-validation is a statistical method used to evaluate the performance of machine learning models. It is a popular technique in the field of data science and is used to assess how well a model generalizes to new, unseen data. The basic idea behind cross-validation is to split the available data into multiple subsets, train the model on some of these subsets, and then evaluate its performance on the remaining subset.
Types of Cross-Validation
There are several different types of cross-validation techniques, each with its own strengths and weaknesses. Some of the most common types include:
- k-Fold Cross-Validation: In k-fold cross-validation, the data is divided into k subsets. The model is trained on k-1 of these subsets and tested on the remaining subset. This process is repeated k times, with each subset used as the test set exactly once. The results are then averaged to obtain a single performance metric.
- Leave-One-Out Cross-Validation (LOOCV): In LOOCV, a single data point is left out as the test set, and the model is trained on the remaining data points. This process is repeated for each data point, and the results are averaged to obtain a final performance metric. LOOCV is computationally expensive but can provide a more accurate estimate of model performance.
- Stratified Cross-Validation: In stratified cross-validation, the data is divided into subsets such that each subset contains approximately the same distribution of the target variable. This helps ensure that the model is trained and tested on a representative sample of the data and can lead to more reliable performance estimates.
Benefits of Cross-Validation
Cross-validation offers several benefits when evaluating machine learning models:
- Model Performance: Cross-validation provides a more reliable estimate of a model's performance compared to a single train-test split. By averaging the results over multiple iterations, cross-validation helps reduce the variability in performance metrics.
- Generalization: Cross-validation helps assess how well a model generalizes to new, unseen data. By testing the model on multiple subsets of the data, cross-validation can provide insights into its ability to make accurate predictions on unseen samples.
- Parameter Tuning: Cross-validation can be used to tune hyperparameters of a model. By evaluating the model's performance on different parameter values across multiple folds, one can identify the optimal set of hyperparameters that maximize performance.
Implementation of Cross-Validation
Implementing cross-validation in practice involves the following steps:
- Data Preprocessing: Prepare the data for cross-validation by cleaning, encoding categorical variables, and scaling numerical features as needed.
- Split Data: Divide the data into training and testing sets. For cross-validation, further split the training set into k folds.
- Model Training and Evaluation: Train the model on k-1 folds of the training data and evaluate its performance on the remaining fold. Repeat this process for each fold to obtain performance metrics for each iteration.
- Average Results: Calculate the average performance metrics across all iterations to obtain a final estimate of the model's performance.
Cross-Validation in Python
Python provides several libraries that make it easy to implement cross-validation, such as scikit-learn. Below is an example of how to perform k-fold cross-validation using scikit-learn:
```python from sklearn.model_selection import KFold from sklearn.linear_model import LogisticRegression from sklearn.datasets import load_iris from sklearn.metrics import accuracy_score # Load the Iris dataset data = load_iris() X = data.data y = data.target # Initialize the KFold class kf = KFold(n_splits=5, shuffle=True, random_state=42) # Initialize the model model = LogisticRegression() # Perform k-fold cross-validation for train_index, test_index in kf.split(X): X_train, X_test = X[train_index], X[test_index] y_train, y_test = y[train_index], y[test_index] # Train the model.
What's Your Reaction?
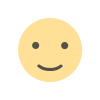
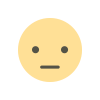
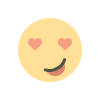
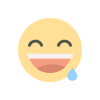
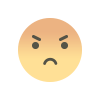
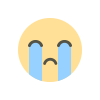
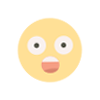