Stochastic Gradient Descent (SGD)
Learn about Stochastic Gradient Descent (SGD) - a popular optimization algorithm for training machine learning models efficiently.
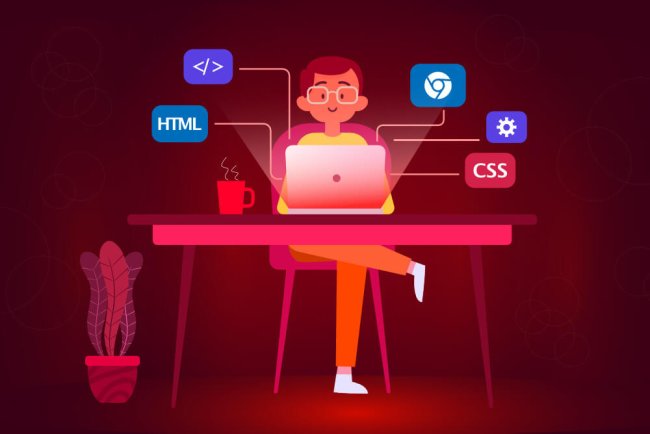
Stochastic Gradient Descent (SGD)
Stochastic Gradient Descent (SGD) is a popular optimization algorithm used in machine learning and deep learning for training models. It is particularly useful when working with large datasets as it processes training examples one at a time rather than in batches, making it more computationally efficient.
Overview
SGD is a variant of the gradient descent optimization algorithm, which is used to minimize a loss function by adjusting the parameters of a model. In traditional gradient descent, the model parameters are updated based on the average gradient of the entire training dataset. However, in stochastic gradient descent, the parameters are updated based on the gradient of a single training example or a small subset of examples (mini-batch).
Algorithm
The basic steps of the stochastic gradient descent algorithm are as follows:
- Initialize the model parameters randomly or with pre-trained values.
- Repeat until convergence or a certain number of iterations:
- Randomly shuffle the training data.
- For each training example:
- Compute the gradient of the loss function with respect to the model parameters.
- Update the model parameters using the gradient descent update rule:
theta = theta - learning_rate * gradient
Benefits of SGD
Some of the key benefits of using stochastic gradient descent include:
- Efficiency: SGD processes training examples individually, making it faster and more memory-efficient than batch gradient descent when working with large datasets.
- Generalization: The stochastic nature of SGD can help prevent overfitting by introducing noise into the parameter updates.
- Robustness: SGD is robust to noisy data and can escape local minima more easily than batch gradient descent.
Hyperparameters
When using stochastic gradient descent, there are several hyperparameters that need to be tuned to achieve optimal performance:
- Learning rate: The step size used to update the model parameters. A high learning rate can cause the algorithm to diverge, while a low learning rate can result in slow convergence.
- Batch size: The number of training examples used to compute each parameter update. A larger batch size can improve convergence stability but may be computationally expensive.
- Number of epochs: The number of times the algorithm iterates over the entire training dataset. Increasing the number of epochs can improve model performance but may lead to overfitting.
Variants of SGD
There are several variants of stochastic gradient descent that aim to improve upon the basic algorithm. Some popular variants include:
- Mini-batch SGD: Combines the efficiency of SGD with the stability of batch gradient descent by updating the model parameters using a small batch of training examples.
- Momentum: Introduces a momentum term to the parameter updates to accelerate convergence and dampen oscillations.
- Adagrad: Adapts the learning rate for each parameter based on the historical gradients, allowing for larger updates for infrequent parameters.
- Adam: Combines the benefits of momentum and Adagrad by using adaptive learning rates and momentum for parameter updates.
Implementation
SGD is commonly implemented in popular machine learning libraries such as TensorFlow, PyTorch, and scikit-learn. Here is a simple example of implementing SGD in Python using scikit-learn:
from sklearn.linear_model import SGDClassifier # Create an instance of the SGDClassifier sgd = SGDClassifier(loss='log', alpha=0.01, max_iter=1000, random_state=42) # Fit the model to the training data sgd.fit(X_train, y_train) # Make predictions on the test data predictions = sgd.predict(X_test)
What's Your Reaction?
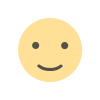
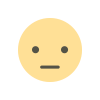
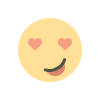
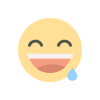
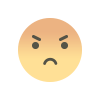
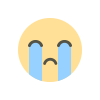
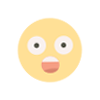