SQL
Learn SQL - the language used to manage and manipulate databases. Master SQL queries, data retrieval, and database design with our comprehensive guide.
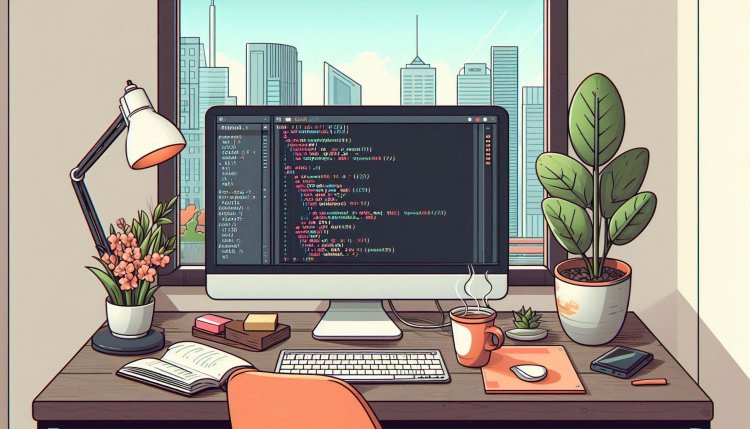
SQL
Here is an example of a SQL query:
SELECT column1, column2
FROM table_name
WHERE condition;
Explanation:
- SELECT: This keyword is used to select data from a database.
- column1, column2: These are the columns you want to retrieve from the database.
- FROM: Specifies the table from which to retrieve the data.
- table_name: The name of the table where the data is stored.
- WHERE: Used to filter records based on a condition.
- condition: The criteria that must be met for a record to be included in the result set.
Example:
SELECT first_name, last_name
FROM employees
WHERE department = 'IT';
This query selects the first name and last name of employees from the employees
table where the department is 'IT'.
SQL Data Types:
Here are some common SQL data types:
Data Type | Description |
---|---|
INT | Integer (whole number) |
VARCHAR(n) | Variable-length character string with a maximum length of n characters |
DATE | Date in the format 'YYYY-MM-DD' |
SQL Joins:
Joins are used to combine rows from two or more tables based on a related column between them. Here are the types of joins:
- INNER JOIN: Returns rows when there is a match in both tables.
- LEFT JOIN: Returns all rows from the left table and the matched rows from the right table.
- RIGHT JOIN: Returns all rows from the right table and the matched rows from the left table.
- FULL JOIN: Returns all rows when there is a match in one of the tables.
Example of a JOIN:
SELECT employees.first_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id;
This query selects the first name of employees and the department name they belong to by joining the employees
table with the departments
table based on the department_id
.
SQL Functions:
SQL provides various functions to perform calculations, manipulate strings, and more. Here are some common SQL functions:
- AVG(): Calculates the average value of a column.
- MAX(): Returns the maximum value in a column.
- MIN(): Returns the minimum value in a column.
- COUNT(): Returns the number of rows that match a specified condition.
Example of using SQL Functions:
SELECT AVG(salary) AS average_salary
FROM employees
WHERE department = 'Finance';
This query calculates the average salary of employees in the 'Finance' department.
What's Your Reaction?
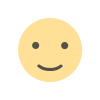
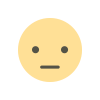
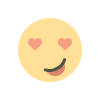
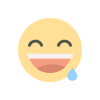
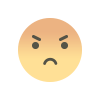
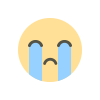
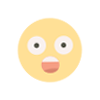