Ruby on Rails
Ruby on Rails is a popular open-source web application framework written in Ruby language. It emphasizes convention over configuration, making web development faster and more efficient.
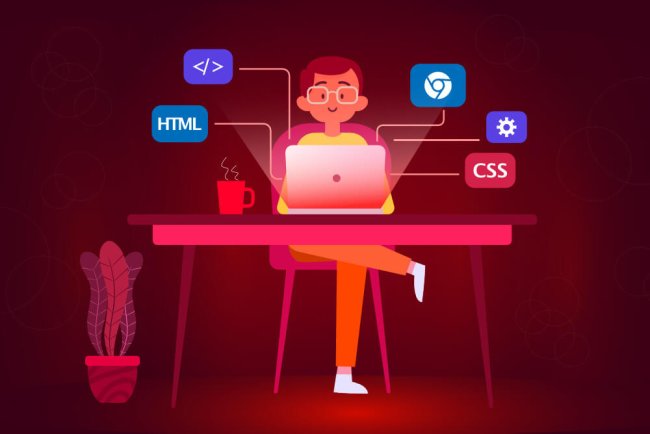
Ruby on Rails
Ruby on Rails, often simply referred to as Rails, is a popular web application framework written in the Ruby programming language. It was created by David Heinemeier Hansson and released as open-source software in 2004. Rails follows the Model-View-Controller (MVC) architectural pattern, which separates the application logic into three interconnected components. In this article, we will explore the key concepts and features of Ruby on Rails in 950 words.
Key Concepts:
1. Convention over Configuration:
Rails follows the principle of "convention over configuration," which means that the framework makes assumptions about how things should be done based on a set of conventions. This minimizes the amount of configuration that developers need to write, allowing them to focus on writing code that matters.
2. DRY (Don't Repeat Yourself):
Rails encourages developers to follow the DRY principle, which emphasizes the importance of writing reusable code. By reducing duplication and promoting code reusability, developers can build applications more efficiently and maintain them more easily.
3. RESTful Routing:
Rails promotes the use of RESTful routing, which maps HTTP verbs (GET, POST, PUT, DELETE) to CRUD (Create, Read, Update, Delete) operations on resources. This makes it easier to design and implement APIs that adhere to RESTful principles.
Key Features:
1. ActiveRecord:
ActiveRecord is Rails' object-relational mapping (ORM) library, which simplifies database interactions by mapping database tables to Ruby objects. With ActiveRecord, developers can perform database operations using Ruby methods, without writing SQL queries manually.
2. Scaffolding:
Rails provides scaffolding generators that can automatically generate code for common tasks such as creating models, controllers, and views. While scaffolding is useful for prototyping, it is important to customize and refine the generated code to meet the specific requirements of the application.
3. Asset Pipeline:
The asset pipeline in Rails manages and processes static assets such as stylesheets, JavaScript files, and images. By concatenating, minifying, and caching assets, the asset pipeline improves the performance and maintainability of web applications.
4. Testing Support:
Rails comes with built-in support for testing, including unit tests, functional tests, and integration tests. Developers can use testing frameworks like RSpec and Capybara to write comprehensive test suites that ensure the reliability and correctness of their code.
5. Security Features:
Rails includes security features such as Cross-Site Scripting (XSS) protection, Cross-Site Request Forgery (CSRF) protection, and SQL injection prevention. By following secure coding practices and leveraging Rails' security features, developers can build web applications that are resistant to common security vulnerabilities.
Advantages of Ruby on Rails:
- Productivity: Rails' conventions and built-in tools enable developers to build web applications quickly and efficiently.
- Community Support: Rails has a large and active community of developers who contribute to the framework and provide support through forums, meetups, and online resources.
- Scalability: Rails is designed to scale with the growth of web applications, making it suitable for both small startups and large enterprises.
- Flexibility: Rails' modular design and extensive ecosystem of gems allow developers to customize and extend the framework to meet diverse requirements.
Example of a Simple Rails Application:
Below is an example of a simple Rails application that displays a list of products:
1. Generate a new Rails application:
```bash rails new myapp cd myapp ```
2. Generate a Product model:
```bash rails generate model Product name:string price:decimal rails db:migrate ```
3. Generate a Products controller:
```bash rails generate controller Products index ```
4. Define the index action in the Products controller:
```ruby class ProductsController < ApplicationController def index @products = Product.all end end ```
What's Your Reaction?
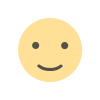
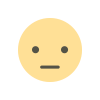
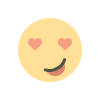
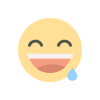
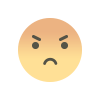
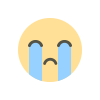
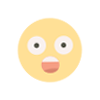