Ruby
Ruby is a dynamic, object-oriented programming language known for its simplicity and productivity. Discover its power and elegance with Ruby.
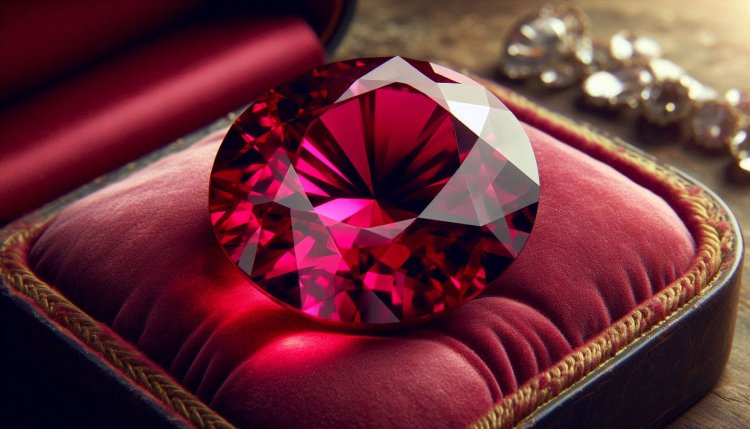
Ruby
Ruby is a dynamic, reflective, object-oriented programming language known for its simplicity and elegance. It was designed and developed by Yukihiro Matsumoto in the mid-1990s with the goal of making programming fun and productive. Ruby draws inspiration from languages like Perl, Smalltalk, Eiffel, Ada, and Lisp.
Key Features of Ruby:
- Simple and Elegant Syntax: Ruby's syntax is clear and easy to read, which makes it a popular choice among developers.
- Object-Oriented: Everything in Ruby is an object, and Ruby follows the object-oriented programming paradigm.
- Dynamically Typed: Ruby is dynamically typed, meaning you don't have to specify the data type of a variable when you declare it.
- Garbage Collection: Ruby has automatic memory management through garbage collection, which helps in managing memory efficiently.
- Meta-Programming: Ruby allows you to write code that can modify or extend itself at runtime, which opens up powerful possibilities for creating flexible and reusable code.
Basic Syntax:
Here is a simple "Hello, World!" program in Ruby:
# This is a comment in Ruby
puts "Hello, World!"
Variables:
Variables in Ruby are declared using the prefix '$', '@', or no prefix at all, depending on the scope of the variable:
# Local variable
name = "Alice"
# Instance variable
@age = 30
# Global variable
$company = "ABC Inc."
Control Structures:
Ruby supports the usual control structures found in most programming languages, such as if-else, while, for, and case:
# If-else statement
if age >= 18
puts "You are an adult."
else
puts "You are a minor."
end
# While loop
counter = 0
while counter < 5
puts counter
counter += 1
end
# For loop
for i in 1..5
puts i
end
# Case statement
grade = "B"
case grade
when "A"
puts "Excellent"
when "B"
puts "Good"
else
puts "Needs Improvement"
end
Methods:
Methods in Ruby are defined using the 'def' keyword:
def greet(name)
puts "Hello, #{name}!"
end
greet("Bob") # Output: Hello, Bob!
Classes and Objects:
Ruby is an object-oriented language, and classes are defined using the 'class' keyword. Here's an example of a simple class in Ruby:
class Person
def initialize(name, age)
@name = name
@age = age
end
def greet
puts "Hello, my name is #{@name} and I am #{@age} years old."
end
end
person = Person.new("Alice", 25)
person.greet
Inheritance:
Ruby supports single inheritance, where a class can inherit from another class using the '<' symbol:
class Student < Person
def initialize(name, age, grade)
super(name, age)
@grade = grade
end
def display_info
puts "#{@name} is a student in grade #{@grade}."
end
end
student = Student.new("Bob", 20, "A")
student.greet
student.display_info
Modules:
Modules in Ruby provide a way to group related methods, classes, and constants together. They can be included in classes to add functionality:
module MathFunctions
def self.square(x)
x * x
end
end
class Calculator
include MathFunctions
end
calc = Calculator.new
puts
What's Your Reaction?
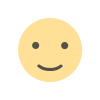
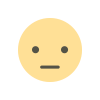
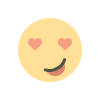
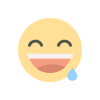
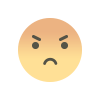
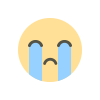
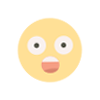