RESTful APIs
Learn about RESTful APIs, their principles, and best practices for building efficient and scalable web services. Find resources and examples here.
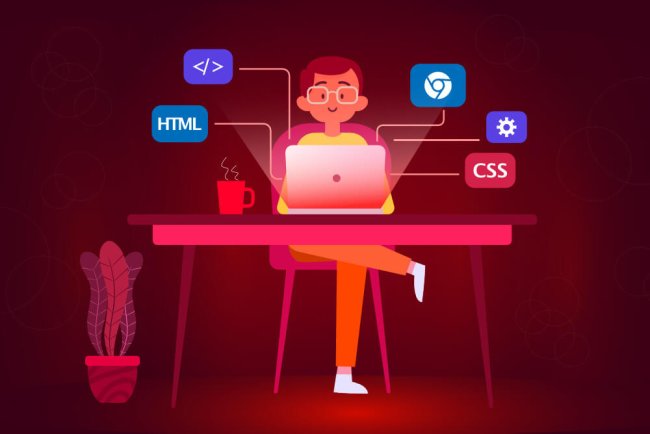
RESTful APIs
Representational State Transfer (REST) is an architectural style for designing networked applications. RESTful APIs adhere to the principles of REST and are designed to be simple, scalable, and stateless. These APIs use standard HTTP methods such as GET, POST, PUT, DELETE to perform CRUD operations (Create, Read, Update, Delete) on resources.
Key Principles of RESTful APIs:
- Resource-Based: Resources are entities that can be accessed and manipulated. Each resource is identified by a unique URI (Uniform Resource Identifier).
- Uniform Interface: The interface for interacting with resources should be uniform and consistent. This includes the use of standard HTTP methods (GET, POST, PUT, DELETE) and status codes (200 OK, 404 Not Found).
- Stateless: Each request from a client to the server should contain all the information needed to fulfill that request. The server should not store any client state between requests.
- Client-Server Architecture: The client and server are separate entities that communicate through a stateless protocol (such as HTTP). This separation allows for better scalability and flexibility.
- Layered System: The architecture should be organized in layers, with each layer having a specific role and responsibility. This allows for better separation of concerns and easier maintenance.
HTTP Methods in RESTful APIs:
RESTful APIs use the following HTTP methods to perform operations on resources:
- GET: Retrieve a resource or a collection of resources.
- POST: Create a new resource.
- PUT: Update an existing resource (replaces the entire resource).
- PATCH: Update an existing resource (partially).
- DELETE: Delete a resource.
Response Codes in RESTful APIs:
HTTP status codes are used to indicate the success or failure of a request in RESTful APIs. Some common status codes include:
- 200 OK: The request was successful.
- 201 Created: The resource was successfully created.
- 400 Bad Request: The request was malformed or invalid.
- 404 Not Found: The requested resource was not found.
- 500 Internal Server Error: An unexpected error occurred on the server.
Authentication and Security in RESTful APIs:
Authentication and security are important considerations when designing RESTful APIs. Common methods for securing APIs include:
- API Keys: Unique identifiers used to authenticate requests.
- OAuth: Open standard for access delegation, allowing secure authorization without sharing credentials.
- HTTPS: Secure communication over the web using SSL/TLS encryption.
- Rate Limiting: Restricting the number of requests a client can make within a given time period to prevent abuse.
Best Practices for Designing RESTful APIs:
When designing RESTful APIs, consider the following best practices:
- Use Nouns for Resource URIs: Use descriptive nouns to represent resources in URIs (e.g., /users, /products).
- Versioning: Include a version number in the API URI to allow for backward compatibility (e.g., /v1/users).
- Use Plural Nouns: Use plural nouns for resource URIs to indicate collections of resources (e.g., /users, /products).
- Use HTTP Headers: Use HTTP headers for metadata and control information (e.g., content type, caching).
- Provide Documentation: Document the API endpoints, request/response formats, and error handling to help.
What's Your Reaction?
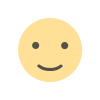
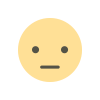
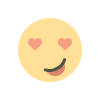
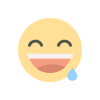
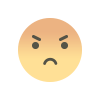
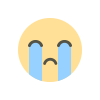
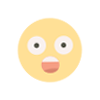