Rectified Linear Unit (ReLU)
Learn about Rectified Linear Unit (ReLU), a popular activation function in neural networks that helps prevent the vanishing gradient problem.
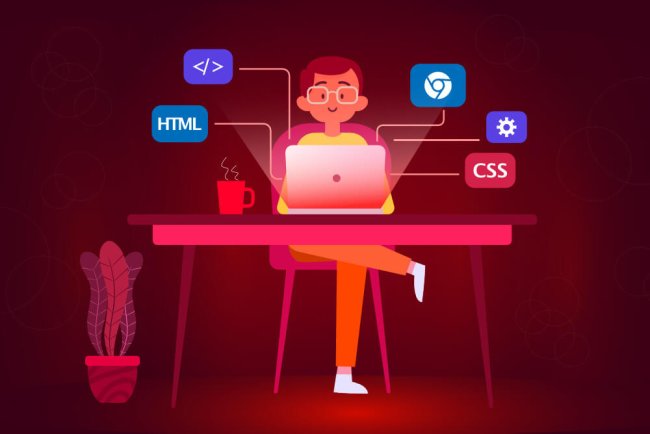
ReLU (Rectified Linear Unit) is a popular activation function used in neural networks, especially in deep learning models. It is a simple but effective way to introduce non-linearity in the network, which helps in capturing complex patterns in the data. In this guide, we will explore the concept of ReLU in detail, its advantages, and how it is implemented in neural networks. ### What is ReLU? ReLU stands for Rectified Linear Unit. It is an activation function that introduces non-linearity in the neural network by replacing all negative values in the input with zero. Mathematically, the ReLU function can be defined as: \[ f(x) = max(0, x) \] Where \( x \) is the input to the function and \( f(x) \) is the output after applying the ReLU activation. ### Advantages of ReLU ReLU has several advantages that make it a popular choice for activation functions in deep learning models: 1. **Simplicity**: ReLU is a simple function that is easy to implement and computationally efficient. It only involves a single operation (max(0, x)) and does not require any additional parameters. 2. **Non-linearity**: ReLU introduces non-linearity in the network, which is essential for capturing complex patterns in the data. The ability to model non-linear relationships is crucial for the success of deep learning models. 3. **Sparse Activation**: ReLU produces sparse activations by setting negative values to zero. This sparsity can help in reducing the computational load and overfitting in the network. 4. **Gradient Descent**: ReLU has a constant gradient of 1 for positive values, which helps in mitigating the vanishing gradient problem during backpropagation. This property makes training deep networks more stable and efficient. 5. **Efficient Training**: ReLU is known to accelerate the training of deep neural networks due to its simple and efficient nature. The non-saturating behavior of ReLU allows for faster convergence of the optimization algorithm. ### Implementing ReLU in Neural Networks ReLU is commonly used as an activation function in the hidden layers of neural networks. When a neural network is fed with input data, the ReLU activation is applied to the output of each neuron in the hidden layers. The ReLU function applies the element-wise max(0, x) operation to the input values, setting all negative values to zero. Here is a simple example of implementing ReLU in a neural network using Python and NumPy: ```python import numpy as np def relu(x): return np.maximum(0, x) # Input data x = np.array([1, -2, 3, -4, 5]) # Applying ReLU activation output = relu(x) print(output) ``` In this example, the `relu` function takes an input array `x` and applies the max(0, x) operation element-wise to produce the output after applying ReLU activation. The output will contain only non-negative values, as the negative values are set to zero. ### Visualizing ReLU Activation Let's visualize the ReLU activation function to understand how it works. We will plot the ReLU function over a range of input values: ```python import matplotlib.pyplot as plt # Define the ReLU function def relu(x): return max(0, x) # Generate input values x = np.linspace(-5, 5, 100) y = [relu(i) for i in x] # Plot the ReLU function plt.figure(figsize=(8, 6)) plt.plot(x, y, label='ReLU', color='b') plt.xlabel('Input') plt.ylabel('Output') plt.title('ReLU Activation Function') plt.grid(True) plt.legend() plt.show() ``` In the plot, you will see that the ReLU function is a linear function for positive values (output = input) and zero for negative values. This behavior introduces non-linearity in the network, allowing it to learn complex patterns in the data. ### Variants of ReLU While the standard ReLU function is widely used, there are several variants of ReLU that have been proposed to address its limitations. Some of the common variants include: 1. **Leaky ReLU**: Leaky ReLU introduces a small slope for negative values instead of setting them to zero. It is defined as: \[ f(x) = max(\alpha x, x) \] where \( \alpha \) is a small positive constant. 2. **Parametric ReLU (PReLU)**: PReLU extends Leaky ReLU by allowing the slope to be learned during training. It introduces an additional parameter that is optimized along with the network weights. 3. **Exponential Linear Unit (ELU)**: ELU is another variant of ReLU that smooths the transition for negative values by using an exponential.
What's Your Reaction?
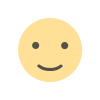
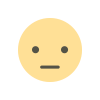
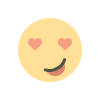
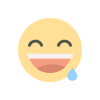
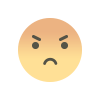
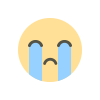
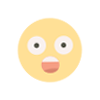