Random Search
Discover new and interesting websites with Random Search. Get a surprise every time you click the button. Start exploring now!
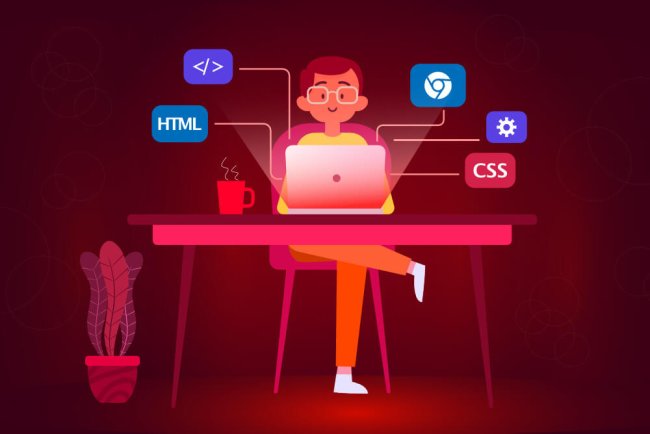
Random Search
Random search is a method used in optimization and machine learning to find solutions to problems by randomly sampling the search space. It is a simple yet effective technique that does not require any prior knowledge of the problem or the search space.
How Random Search Works
In random search, a random solution is generated in the search space, and its performance is evaluated using a predefined objective function. This process is repeated for a specified number of iterations or until a stopping criterion is met.
Unlike other optimization techniques that rely on gradients or heuristics, random search does not make any assumptions about the problem structure. It explores the search space randomly, which can sometimes lead to finding better solutions than other more sophisticated methods.
Advantages of Random Search
- Simplicity: Random search is easy to implement and does not require complex algorithms or heuristics.
- Exploration: Random search can explore the search space more thoroughly compared to gradient-based methods that may get stuck in local optima.
- Robustness: Random search is robust to noise and can handle noisy objective functions effectively.
- Parallelization: Random search can be easily parallelized by running multiple random searches concurrently.
Applications of Random Search
Random search is used in various fields where optimization is required, such as:
- Parameter tuning in machine learning algorithms
- Hyperparameter optimization in deep learning models
- Optimization of simulation models in engineering and science
- Exploration of design spaces in architecture and product design
Comparison with Grid Search
Random search is often compared with grid search, another popular optimization technique. While grid search evaluates solutions on a predefined grid of parameter values, random search samples solutions randomly from the search space.
Grid search can be computationally expensive, especially in high-dimensional search spaces, as it evaluates all possible combinations of parameter values. Random search, on the other hand, can be more efficient in exploring the search space without the need for an exhaustive search.
Implementing Random Search in Python
Here is an example of how to implement random search for hyperparameter optimization in a machine learning model using Python and scikit-learn:
```python from sklearn.model_selection import RandomizedSearchCV from sklearn.ensemble import RandomForestClassifier from scipy.stats import randint # Define the parameter grid param_dist = { 'n_estimators': randint(10, 100), 'max_depth': randint(1, 10), 'min_samples_split': randint(2, 20) } # Create a random search object random_search = RandomizedSearchCV(RandomForestClassifier(), param_distributions=param_dist, n_iter=10) # Fit the random search object random_search.fit(X_train, y_train) # Get the best parameters best_params = random_search.best_params_ ```
Conclusion
Random search is a simple yet powerful optimization technique that can be used in various domains to find solutions to complex problems. Its ability to explore the search space randomly makes it a valuable tool for optimization tasks where the problem structure is unknown or complex.
By leveraging the advantages of random search, researchers and practitioners can efficiently optimize parameters and models in machine learning, engineering, and other fields, leading to improved performance and better solutions.
What's Your Reaction?
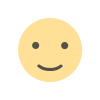
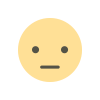
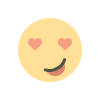
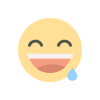
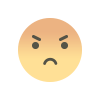
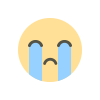
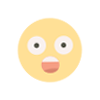