Python
Python is a versatile and powerful programming language used for web development, data analysis, artificial intelligence, and more. Learn Python today!
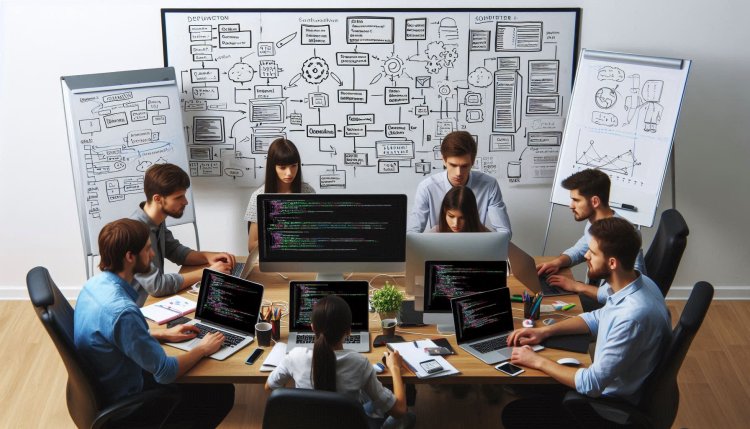
Python Programming Language
Python is a high-level, interpreted programming language known for its simplicity and readability. It was created by Guido van Rossum and first released in 1991. Python is widely used in various fields such as web development, data science, artificial intelligence, and more.
Features of Python
- Easy to Learn: Python has a simple and clean syntax, making it easy for beginners to pick up and start coding.
- Interpreted Language: Python code is executed line by line by the Python interpreter, making it easy to test and debug code.
- High-Level Language: Python abstracts low-level details, allowing programmers to focus on solving problems rather than worrying about memory management or other technical details.
- Dynamic Typing: Python is dynamically typed, meaning you don't have to declare variable types. This makes coding faster and more flexible.
- Extensive Standard Library: Python comes with a rich standard library that provides pre-written modules and functions for various tasks.
Example Python Code
Here is a simple "Hello, World!" program written in Python:
print("Hello, World!")
Data Types in Python
Python supports various data types, including:
- Integers: whole numbers such as 1, 10, -5
- Floats: decimal numbers such as 3.14, 2.718
- Strings: sequences of characters enclosed in single or double quotes, such as "hello", 'python'
- Lists: ordered collections of items that can be of different types
- Dictionaries: key-value pairs that allow you to store and retrieve data based on keys
- Tuples: immutable ordered collections of items
- Sets: unordered collections of unique items
- Boolean: True or False values
Control Flow in Python
Python provides various control flow statements to control the flow of execution in a program:
- If-Else Statements: Execute different blocks of code based on conditions
- For Loops: Iterate over a sequence of items
- While Loops: Repeat a block of code while a condition is true
- Break and Continue: Control the flow within loops
Functions in Python
Functions in Python allow you to encapsulate reusable pieces of code. Here is an example of a simple function that adds two numbers:
def add_numbers(a, b):
return a + b
result = add_numbers(5, 3)
print(result) # Output: 8
Modules and Packages
Python allows you to organize code into modules and packages for better code organization and reusability. Modules are files containing Python code, while packages are directories containing multiple modules.
Object-Oriented Programming in Python
Python supports object-oriented programming (OOP) concepts such as classes, objects, inheritance, and polymorphism. Here is an example of a simple class in Python:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print("Hello, my name is", self.name)
# Create an object of the Person class
person1 = Person("Alice", 30)
person1.greet() # Output: Hello, my name is Alice
Exception Handling
Python allows you to handle exceptions using try-except blocks to gracefully handle errors in your code:
try:
result = 10 / 0
except
What's Your Reaction?
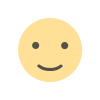
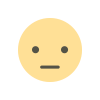
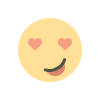
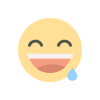
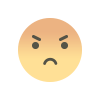
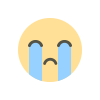
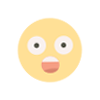