Huber Loss
Learn about Huber Loss, a robust regression loss function that combines the best of Mean Absolute Error and Mean Squared Error for more stable optimization.
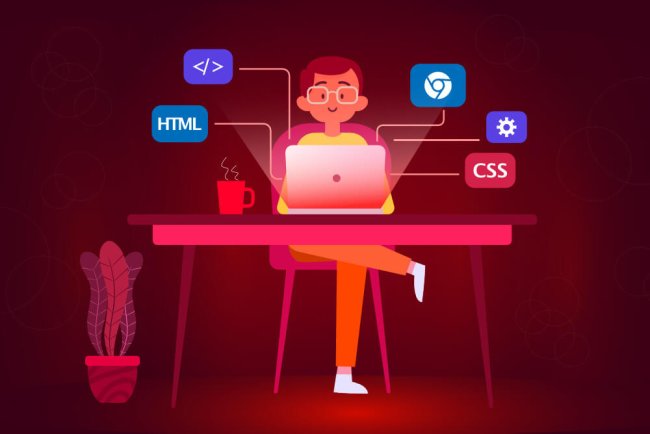
Huber Loss
The Huber loss function is a loss function used in regression tasks. It is less sensitive to outliers in the data compared to the mean squared error (MSE) loss function. The Huber loss function combines the best properties of the mean absolute error (MAE) and the mean squared error loss functions.
Mathematical Formulation
The Huber loss function is defined as:
\[ L_{\delta}(y, f(x)) = \begin{cases} \frac{1}{2}(y - f(x))^2 & \text{for } |y - f(x)| \leq \delta \\ \delta(|y - f(x)| - \frac{1}{2}\delta) & \text{otherwise} \end{cases} \]
Here, \( y \) represents the true target value, \( f(x) \) represents the predicted value by the model, and \( \delta \) is a hyperparameter that determines the point at which the loss function transitions from quadratic to linear behavior.
When the absolute difference between the true target value and the predicted value is less than or equal to \( \delta \), the loss function is quadratic, similar to the mean squared error loss. When the absolute difference exceeds \( \delta \), the loss function becomes linear, similar to the mean absolute error loss.
Advantages of Huber Loss
The Huber loss function has several advantages:
- Robustness to Outliers: The Huber loss function is less sensitive to outliers in the data compared to the mean squared error loss. This is because the loss function becomes linear for large errors, which reduces the impact of outliers on the overall loss.
- Smoothness: The Huber loss function is a smooth function that is differentiable everywhere, unlike the mean absolute error loss, which is not differentiable at zero.
- Trade-off between MAE and MSE: The Huber loss function provides a trade-off between the mean absolute error and the mean squared error loss functions. It penalizes large errors like the mean squared error loss while maintaining the robustness to outliers like the mean absolute error loss.
Implementation in Python
Here is an example of how to implement the Huber loss function in Python:
```python import numpy as np def huber_loss(y_true, y_pred, delta=1.0): error = np.abs(y_true - y_pred) quadratic_part = np.minimum(error, delta) linear_part = error - quadratic_part return 0.5 * quadratic_part**2 + delta * linear_part # Example usage y_true = np.array([1, 2, 3, 4, 5]) y_pred = np.array([1.5, 2.5, 2.8, 3.9, 4.2]) loss = huber_loss(y_true, y_pred, delta=1.0) print("Huber Loss:", loss) ```
In this Python implementation, the function `huber_loss` calculates the Huber loss given the true target values `y_true`, predicted values `y_pred`, and the hyperparameter `delta`. It first calculates the absolute error between the true and predicted values, then splits the error into quadratic and linear parts based on the value of `delta`, and returns the Huber loss.
Applications
The Huber loss function is commonly used in regression tasks where the data may contain outliers or noise. Some common applications of the Huber loss function include:
- Robotic Control: In robotics, the Huber loss function can be used to train models for controlling robotic arms or vehicles, where outliers or noisy sensor data may be present.
- Financial Forecasting: In finance, the Huber loss function can be used to predict stock prices or financial indicators, where unexpected events or anomalies can lead to outliers in the data.
What's Your Reaction?
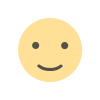
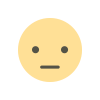
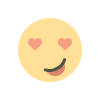
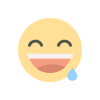
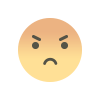
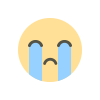
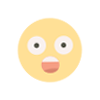