GraphQL
Learn how to query and manipulate data efficiently with GraphQL. Simplify your API development process with this powerful query language.
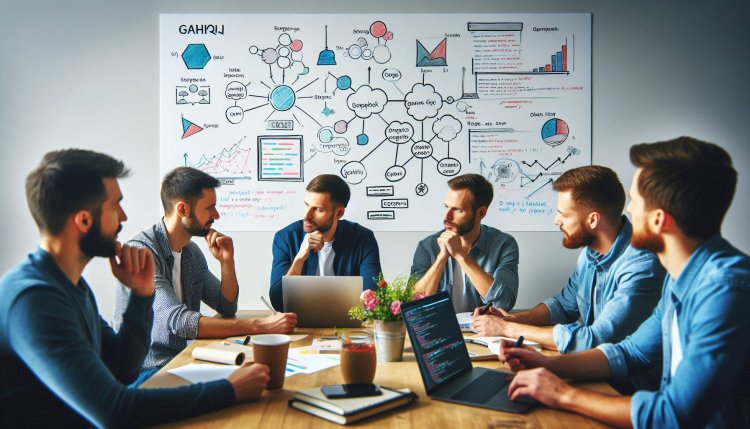
GraphQL
GraphQL is a query language for your API and a runtime for executing those queries by using a type system you define for your data. It was developed by Facebook in 2012 and released as an open-source project in 2015.
Why GraphQL?
GraphQL offers several advantages over traditional REST APIs:
- Efficient data fetching: With GraphQL, clients can request only the data they need, reducing over-fetching and under-fetching issues commonly seen in REST APIs.
- Single endpoint: GraphQL APIs have a single endpoint for all data queries, making it easier to manage and allowing clients to request multiple resources in a single request.
- Strongly typed schema: GraphQL APIs are defined by a schema that specifies the types of data available and the relationships between them, providing clear documentation and validation.
- Introspection: Clients can query the schema itself to discover available types and fields, enabling powerful tools like auto-completion and documentation generation.
Basic Concepts
There are several key concepts in GraphQL:
- Schema: The schema defines the types available in the API and the relationships between them. It consists of object types, scalar types, input types, interfaces, unions, and enums.
- Queries: Queries are used to request data from the API. They specify the fields and relationships to be included in the response.
- Mutations: Mutations are used to modify data on the server. They can create, update, or delete data and are typically performed using POST requests.
- Subscriptions: Subscriptions allow clients to receive real-time updates from the server. They are typically used for implementing features like live chat or notifications.
Example
Here is a simple example of a GraphQL query:
query { user(id: 123) { name email posts { title content } } }
This query requests the name and email of a user with ID 123, as well as the titles and content of their posts. The response will only include the requested fields, avoiding over-fetching of data.
Tools and Libraries
There are several tools and libraries available for working with GraphQL:
- GraphQL Playground: An interactive GraphQL IDE that allows you to explore your API, write and execute queries, and view the results.
- Apollo Client: A comprehensive GraphQL client that integrates seamlessly with React, Angular, and Vue.js applications.
- graphql-js: The reference implementation of GraphQL in JavaScript, used for building GraphQL servers and executing queries.
- GraphiQL: An in-browser IDE for exploring GraphQL APIs, similar to GraphQL Playground.
Best Practices
When working with GraphQL, consider the following best practices:
- Keep your schema simple: Avoid creating overly complex schemas with deep nesting and circular references. Keep your types and fields simple and focused on specific use cases.
- Use field aliases: Field aliases allow clients to request the same field multiple times with different arguments, enabling more flexible queries.
- Implement pagination: When querying lists of data, use pagination to limit the number of results returned and provide efficient navigation through large datasets.
- Optimize resolvers: Ensure that your resolver functions are efficient and avoid unnecessary database queries or computations to improve performance.
Conclusion
GraphQL offers a modern approach to building APIs that provides flexibility, efficiency, and powerful tooling for both clients and servers. By defining a clear schema and allowing clients to request only the data they need, GraphQL enables developers to build scalable and maintainable applications.
Whether you are building a new API from scratch or considering transitioning from a REST API, GraphQL is worth exploring for its benefits in data fetching, type safety, and real-time capabilities.
What's Your Reaction?
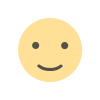
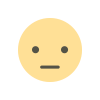
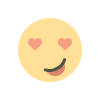
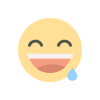
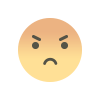
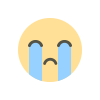
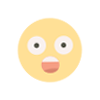