Flask
A lightweight and versatile web framework for Python, Flask allows you to quickly build web applications with ease.
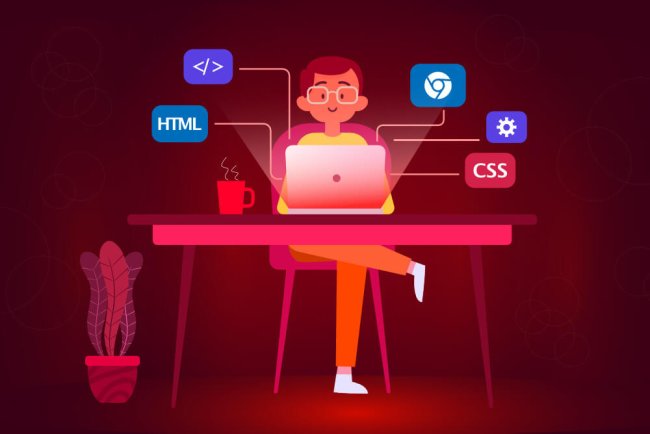
Flask
Flask is a lightweight web application framework written in Python. It is designed to make getting started with web development easy and quick, with minimal boilerplate code.
1. Installation
To install Flask, you can use pip, the Python package manager. Simply run:
pip install Flask
This will install Flask and its dependencies on your system.
2. Hello World
Creating a basic "Hello, World!" application in Flask is simple. Here's a minimal example:
```python from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run() ```
Save this code in a file named app.py
and run it. You should see "Hello, World!" displayed when you visit http://localhost:5000
in your browser.
3. Routing
Flask uses decorators to define routes. Routes are the URLs that the application responds to. In the example above, @app.route('/')
defines the route for the root URL. You can define multiple routes in your application.
4. Templates
Flask supports Jinja2 templates for rendering dynamic content. Templates allow you to separate the presentation layer from the business logic. Here's an example that renders a template:
```python from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): return render_template('index.html', title='Home', content='Welcome to my website!') if __name__ == '__main__': app.run() ```
Create a templates
folder in the same directory as your application and save the template as index.html
inside it.
5. Request and Response
Flask provides access to request and response objects. You can access request data and set response headers. Here's an example that reads query parameters from the request and sets a custom response header:
```python from flask import Flask, request, make_response app = Flask(__name__) @app.route('/') def query_param(): name = request.args.get('name', 'Guest') response = make_response('Hello, ' + name) response.headers['X-Hello'] = 'World' return response if __name__ == '__main__': app.run() ```
6. Static Files
Flask can serve static files such as CSS, JavaScript, and images. Create a static
folder in the same directory as your application and place your static files inside it. You can link to these files in your templates using the url_for()
function.
7. Forms
Flask makes it easy to work with HTML forms. You can define forms using the WTForms
library and handle form submission in your routes. Here's an example:
```python from flask import Flask, render_template, request from flask_wtf import FlaskForm from wtforms import StringField, SubmitField app = Flask(__name__) app.config['SECRET_KEY'] = 'supersecretkey' class NameForm(FlaskForm): name = StringField('Name') submit = SubmitField('Submit') @app.route('/', methods=['GET', 'POST']) def form_example(): form = NameForm() if form.validate_on_submit(): return 'Hello, ' + form.name.data return render_template('form.html', form=form) if __name__ == '__main__': app.run() ```
8. Error Handling
Flask provides ways to handle errors in your application. You can define error handlers for different HTTP status codes or exceptions. Here's an example of handling a 404 error:
```python from flask import Flask, render_template app = Flask(__name__) @app.errorhandler(404) def page_not_found(error): return render_template('404.html'), 404 if __name__ == '__main__': app.run() ```..
What's Your Reaction?
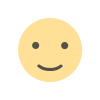
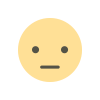
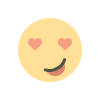
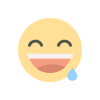
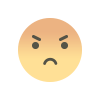
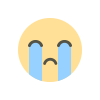
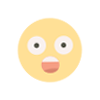