Express.js
Express.js is a fast, unopinionated, and minimalist web framework for Node.js. Build robust and scalable web applications easily with Express.js.
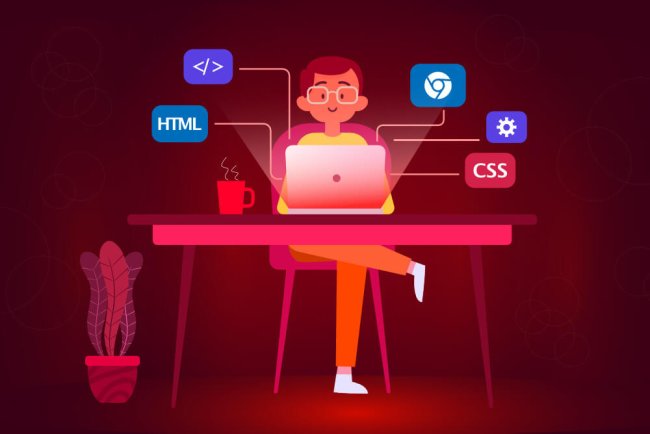
Express.js
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It is designed for building web applications and APIs quickly with minimal effort. Express.js simplifies the process of building server-side applications with Node.js by providing a thin layer of fundamental web application features, without obscuring Node.js features.
Key Features of Express.js:
1. Routing: Express.js provides a simple and intuitive way to define routes for handling different HTTP requests. Routes are defined with HTTP methods like GET, POST, PUT, DELETE, etc. and corresponding handler functions.
2. Middleware: Middleware functions in Express.js are functions that have access to the request and response objects. They can execute any code, make changes to the request and response objects, end the request-response cycle, and call the next middleware function in the stack.
3. Template Engines: Express.js supports several template engines like EJS, Pug, Handlebars, etc. that allow you to generate dynamic HTML content on the server and render it in the browser.
4. Static File Serving: Express.js allows you to serve static files like images, CSS, and JavaScript files by using the built-in middleware function express.static()
.
5. Error Handling: Express.js provides a robust error handling mechanism that allows you to define error-handling middleware functions to handle errors that occur during the request-response cycle.
Creating an Express.js Application:
To create an Express.js application, you need to follow these steps:
- Install Node.js on your system.
- Create a new directory for your application and navigate into it.
- Run
npm init
to create a newpackage.json
file for your application. - Install Express.js by running
npm install express
. - Create an entry file (e.g.,
app.js
) and require Express.js in it. - Create routes, middleware functions, and other necessary components for your application.
- Start the Express.js server using
app.listen()
method.
Example of an Express.js Application:
Here is an example of a simple Express.js application that demonstrates routing and middleware:
```javascript const express = require('express'); const app = express(); // Middleware function app.use((req, res, next) => { console.log('Middleware function executed.'); next(); }); // Route with handler function app.get('/', (req, res) => { res.send('Hello, Express.js!'); }); // Error handling middleware function app.use((err, req, res, next) => { console.error(err); res.status(500).send('Internal Server Error'); }); app.listen(3000, () => { console.log('Server started on port 3000'); }); ```
This example creates an Express.js application with a middleware function that logs a message to the console, a route that responds with a simple message, and an error-handling middleware function.
Express.js Routing:
Express.js routing allows you to define multiple routes with different HTTP methods and handle them using handler functions. Routes can have parameters and can be nested to create more complex routing structures.
Here is an example of defining routes in Express.js:
```javascript app.get('/', (req, res) => { res.send('Home Page'); }); app.post('/users', (req, res) => { res.send('User Created'); }); app.get('/users/:id', (req, res) => { const userId = req.params.id; res.send(`User ID: ${userId}`); }); ```
In this example, we have defined routes for the home page, creating users, and getting a specific user by ID. The user ID is accessed using the req.params
object.
What's Your Reaction?
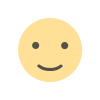
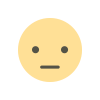
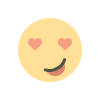
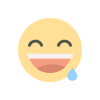
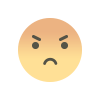
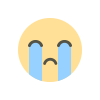
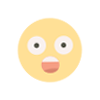