Django
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. Build web applications with ease.
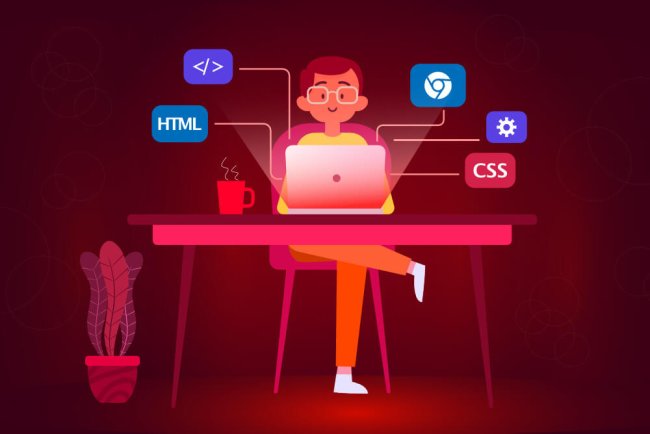
Django Framework
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It follows the DRY (Don't Repeat Yourself) principle and focuses on automating as much as possible to help developers build web applications quickly and efficiently.
Key Features of Django
- MTV Architecture: Django follows the Model-Template-View (MTV) architecture, which helps in separating the different components of a web application.
- ORM: Django comes with a powerful Object-Relational Mapping (ORM) system that allows developers to interact with the database using Python objects.
- Admin Interface: Django provides an admin interface that can be used to manage the application's data without writing any code.
- URL Routing: Django uses a URL dispatcher to route incoming web requests to the appropriate view functions.
- Template Engine: Django has a built-in template engine that allows developers to create dynamic HTML templates.
- Form Handling: Django provides a form handling library that simplifies the process of validating and processing user input.
- Security Features: Django comes with built-in security features such as protection against SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
Getting Started with Django
To start a new Django project, you can use the following command:
django-admin startproject projectname
This will create a new Django project with the given project name. You can then navigate into the project directory and start the development server using the following command:
python manage.py runserver
This will start the development server at http://127.0.0.1:8000/
. You can access your Django application by visiting this URL in a web browser.
Creating Django Apps
In Django, an app is a web application that does something - e.g., a blog, a poll, a forum, etc. To create a new app in Django, you can use the following command:
python manage.py startapp appname
This will create a new Django app with the given app name. You can then define models, views, and templates for your app to implement its functionality.
Defining Models
In Django, models are Python classes that represent database tables. You can define models in your Django app by creating a models.py
file and defining your model classes inside it. Here's an example of a simple model in Django:
```python from django.db import models class Product(models.Model): name = models.CharField(max_length=100) price = models.DecimalField(max_digits=10, decimal_places=2) description = models.TextField() ```
After defining your models, you can create database tables by running the following command:
python manage.py makemigrations
python manage.py migrate
Creating Views
In Django, views are Python functions that take a web request and return a web response. You can define views in your Django app by creating a views.py
file and defining your view functions inside it. Here's an example of a simple view in Django:
```python from django.http import HttpResponse def hello(request): return HttpResponse("Hello, World!") ```
You can then map your views to URLs by defining URL patterns in your Django project's urls.py
file.
Creating Templates
In Django, templates are HTML files that are used to generate the final web page that is sent to the user's browser. You can create templates in your Django app by creating a templates
directory and placing your HTML template files inside it. Here's an example of a simple template in Django:
```html
Hello, World!
```
You can then render your templates in views using Django's template engine to generate dynamic web pages.
Admin Interface
Django provides a built-in admin
What's Your Reaction?
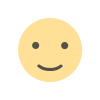
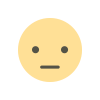
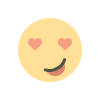
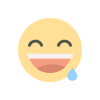
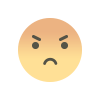
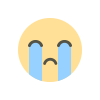
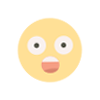