Design patterns in software development
Learn how to efficiently solve common design problems in software development with design patterns. Enhance your coding skills and create robust applications.
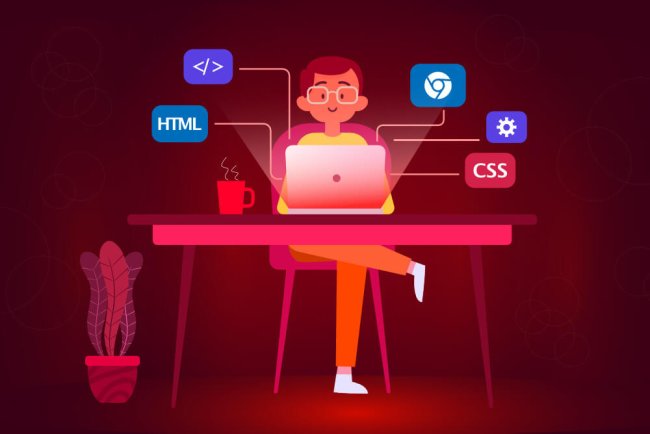
Design Patterns in Software Development
Design patterns are reusable solutions to common problems encountered in software design. They provide a template for solving similar design issues and help developers create flexible and maintainable code. Design patterns are categorized into three main types: creational, structural, and behavioral patterns.
Creational Patterns
Creational patterns focus on object creation mechanisms, providing flexibility in object instantiation. Some common creational patterns include:
- Singleton Pattern: Ensures a class has only one instance and provides a global point of access to that instance.
- Factory Method Pattern: Defines an interface for creating objects but allows subclasses to alter the type of objects that will be created.
- Abstract Factory Pattern: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Builder Pattern: Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
- Prototype Pattern: Creates new objects by copying an existing object, allowing the creation of new instances without specifying the exact class of the object to be copied.
Structural Patterns
Structural patterns focus on object composition and provide ways to simplify the relationships between objects. Some common structural patterns include:
- Adapter Pattern: Allows incompatible interfaces to work together by creating a bridge between them.
- Decorator Pattern: Adds new functionality to an object dynamically without changing its structure.
- Facade Pattern: Provides a unified interface to a set of interfaces in a subsystem, simplifying the interactions for the client.
- Composite Pattern: Composes objects into tree structures to represent part-whole hierarchies.
- Proxy Pattern: Controls access to an object by acting as a surrogate or placeholder.
Behavioral Patterns
Behavioral patterns focus on communication between objects and provide solutions for the interaction between them. Some common behavioral patterns include:
- Observer Pattern: Defines a one-to-many dependency between objects, so that when one object changes state, all its dependents are notified and updated automatically.
- Strategy Pattern: Defines a family of algorithms, encapsulates each one, and makes them interchangeable. Clients can choose the algorithm that suits them at runtime.
- Chain of Responsibility Pattern: Allows multiple objects to handle a request without the sender needing to know the receiver explicitly.
- Template Method Pattern: Defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps of the algorithm without changing its structure.
- Command Pattern: Encapsulates a request as an object, allowing parameterization of clients with different requests, queuing requests, and logging requests.
Benefits of Design Patterns
Design patterns offer several benefits in software development:
- Reusability: Design patterns provide proven solutions to common problems, making it easier to reuse code and design principles.
- Maintainability: By following established design patterns, developers can create code that is easier to maintain and modify over time.
- Scalability: Design patterns help in building scalable software systems that can adapt to changing requirements and grow with the business.
- Abstraction: Design patterns abstract complex design concepts into simple solutions, making it easier for developers to understand and implement them.
- Collaboration: Design patterns provide a common language for developers to communicate and collaborate on design decisions, leading to more cohesive and efficient development teams.
What's Your Reaction?
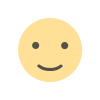
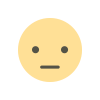
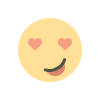
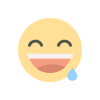
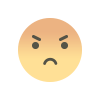
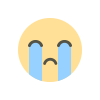
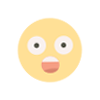