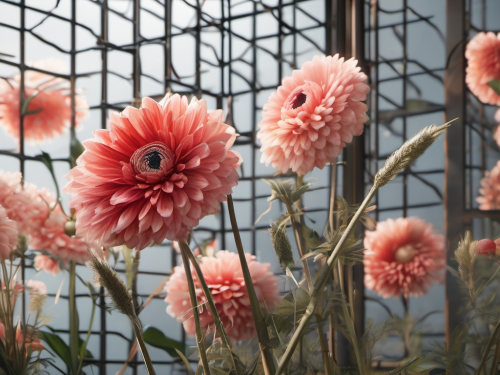
Grid search is a technique used in machine learning to find the optimal hyperparameters for a given model. Hyperparameters are the parameters that are set before the learning process begins, such as the learning rate in a neural network or the number of trees in a random forest. Grid search is a brute force method that involves searching through a specified set of hyperparameters and finding the combination that results in the best performance of the model.
Grid search works by defining a grid of hyperparameters to search through. For example, if we are tuning the hyperparameters of a support vector machine (SVM), we may want to search through different values of the C parameter and the kernel parameter. We would define a grid with different values for C and kernel, such as [0.1, 1, 10] for C and ['linear', 'rbf'] for kernel.
Grid search then trains the model using each combination of hyperparameters in the grid and evaluates the performance using a validation set. The hyperparameters that result in the best performance on the validation set are then selected as the optimal hyperparameters for the model.
Grid search is a simple and straightforward method for hyperparameter tuning that is easy to implement and understand. It allows for an exhaustive search through a specified set of hyperparameters, ensuring that the best combination is found. Grid search is also easily parallelizable, as each combination of hyperparameters can be trained independently.
One of the main limitations of grid search is that it can be computationally expensive, especially when searching through a large number of hyperparameters or when the training time for the model is high. Grid search also does not take into account the interactions between hyperparameters, as it treats each hyperparameter independently. This can lead to suboptimal results if certain hyperparameters are dependent on each other.
Let's consider an example where we want to tune the hyperparameters of a random forest classifier using grid search. We define a grid with different values for the number of trees and the maximum depth of the trees:
param_grid = { 'n_estimators': [50, 100, 200], 'max_depth': [None, 10, 20] }
We then use grid search to find the optimal hyperparameters:
from sklearn.ensemble import RandomForestClassifier from sklearn.model_selection import GridSearchCV rf = RandomForestClassifier() grid_search = GridSearchCV(estimator=rf, param_grid=param_grid, cv=5) grid_search.fit(X_train, y_train)
After fitting the grid search object to the training data, we can access the best hyperparameters and the best score:
best_params = grid_search.best_params_ best_score = grid_search.best_score_
The best_params variable would contain the optimal values for 'n_estimators' and 'max_depth', while the best_score variable would contain the accuracy score of the model with the optimal hyperparameters.
Grid search is a useful technique for hyperparameter tuning in machine learning that can help improve the performance of a model. While it has its limitations, such as being computationally expensive, grid search provides a systematic way to search through different hyperparameters and find the best combination for a given model. By carefully defining the grid of hyperparameters and evaluating the performance of each combination, grid search can help optimize the hyperparameters of a model and improve its predictive accuracy.